template<UnsignedInt dimensions>
Flat class
Flat shader.
Contents
Draws whole mesh with given unshaded color or texture. For colored mesh you need to provide Position attribute in your triangle mesh and call at least setTransformationProjectionMatrix() and setColor().
If you want to use texture, you need to provide also TextureCoordinates attribute. Pass Flag::
For coloring the texture based on intensity you can use the Vector shader.
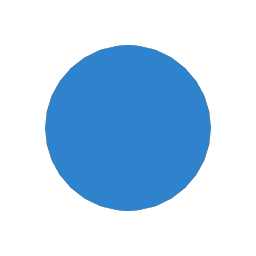
Example usage
Colored mesh
Common mesh setup:
struct Vertex { Vector3 position; }; Vertex data[60]{ //... }; GL::Buffer vertices; vertices.setData(data, GL::BufferUsage::StaticDraw); GL::Mesh mesh; mesh.addVertexBuffer(vertices, 0, Shaders::Flat3D::Position{}) // ... ;
Common rendering setup:
Matrix4 transformationMatrix = Matrix4::translation(Vector3::zAxis(-5.0f)); Matrix4 projectionMatrix = Matrix4::perspectiveProjection(35.0_degf, 1.0f, 0.001f, 100.0f); Shaders::Flat3D shader; shader.setColor(0x2f83cc_rgbf) .setTransformationProjectionMatrix(projectionMatrix*transformationMatrix); mesh.draw(shader);
Textured mesh
Common mesh setup:
struct Vertex { Vector3 position; Vector2 textureCoordinates; }; Vertex data[60]{ // ... }; GL::Buffer vertices; vertices.setData(data, GL::BufferUsage::StaticDraw); GL::Mesh mesh; mesh.addVertexBuffer(vertices, 0, Shaders::Flat3D::Position{}, Shaders::Flat3D::TextureCoordinates{}) // ... ;
Common rendering setup:
Matrix4 transformationMatrix, projectionMatrix; GL::Texture2D texture; Shaders::Flat3D shader{Shaders::Flat3D::Flag::Textured}; shader.setTransformationProjectionMatrix(projectionMatrix*transformationMatrix) .bindTexture(texture); mesh.draw(shader);
Base classes
- class Magnum::GL::AbstractShaderProgram
- Base for shader program implementations.
Public types
- enum class Flag: UnsignedByte { Textured = 1 << 0 }
- Flag.
- using Position = Generic<dimensions>::Position
- Vertex position.
- using TextureCoordinates = Generic<dimensions>::TextureCoordinates
- 2D texture coordinates
-
using Flags = Containers::
EnumSet<Flag> - Flags.
Constructors, destructors, conversion operators
Public functions
- auto flags() const -> Flags
- Flags.
- auto setTransformationProjectionMatrix(const MatrixTypeFor<dimensions, Float>& matrix) -> Flat<dimensions>&
- Set transformation and projection matrix.
- auto setColor(const Color4& color) -> Flat<dimensions>&
- Set color.
-
auto bindTexture(GL::
Texture2D& texture) -> Flat<dimensions>& - Bind texture.
-
auto setTexture(GL::
Texture2D& texture) -> Flat<dimensions>& deprecated - Bind texture.
Enum documentation
template<UnsignedInt dimensions>
enum class Magnum:: Shaders:: Flat<dimensions>:: Flag: UnsignedByte
Flag.
Enumerators | |
---|---|
Textured |
The shader uses texture instead of color |
Typedef documentation
template<UnsignedInt dimensions>
typedef Generic<dimensions>::Position Magnum:: Shaders:: Flat<dimensions>:: Position
Vertex position.
Generic attribute, Vector2 in 2D, Vector3 in 3D.
template<UnsignedInt dimensions>
typedef Generic<dimensions>::TextureCoordinates Magnum:: Shaders:: Flat<dimensions>:: TextureCoordinates
2D texture coordinates
Generic attribute, Vector2. Used only if Flag::
template<UnsignedInt dimensions>
typedef Containers:: EnumSet<Flag> Magnum:: Shaders:: Flat<dimensions>:: Flags
Flags.
Function documentation
template<UnsignedInt dimensions>
Magnum:: Shaders:: Flat<dimensions>:: Flat(Flags flags = {}) explicit
Constructor.
Parameters | |
---|---|
flags | Flags |
template<UnsignedInt dimensions>
Magnum:: Shaders:: Flat<dimensions>:: Flat(NoCreateT) explicit noexcept
Construct without creating the underlying OpenGL object.
The constructed instance is equivalent to moved-from state. Useful in cases where you will overwrite the instance later anyway. Move another object over it to make it useful.
This function can be safely used for constructing (and later destructing) objects even without any OpenGL context being active.
template<UnsignedInt dimensions>
Flat<dimensions>& Magnum:: Shaders:: Flat<dimensions>:: setTransformationProjectionMatrix(const MatrixTypeFor<dimensions, Float>& matrix)
Set transformation and projection matrix.
Returns | Reference to self (for method chaining) |
---|
template<UnsignedInt dimensions>
Flat<dimensions>& Magnum:: Shaders:: Flat<dimensions>:: setColor(const Color4& color)
Set color.
Returns | Reference to self (for method chaining) |
---|
If Flag::0xffffffff_rgbaf
and the color will be multiplied with texture.
template<UnsignedInt dimensions>
Flat<dimensions>& Magnum:: Shaders:: Flat<dimensions>:: bindTexture(GL:: Texture2D& texture)
Bind texture.
Returns | Reference to self (for method chaining) |
---|
Has effect only if Flag::
template<UnsignedInt dimensions>
Flat<dimensions>& Magnum:: Shaders:: Flat<dimensions>:: setTexture(GL:: Texture2D& texture)
Bind texture.