Getting started
Get started with Magnum in matter of minutes.
Contents
Setting up a new project can be pretty gruesome and nobody likes repeating the same process every time. Magnum provides "bootstrap" project structures for many use cases, helping you get up and running in no time.
Download the bootstrap project
The bootstrap repository is located on GitHub. The master
branch contains just a README file and the actual bootstrap projects are in various other branches, each covering some particular use case. For the first project you need the base
branch, which contains only the essential files. Download the branch as an archive and extract it somewhere. Do it rather than cloning the full repository, as it's better to init your new project from scratch with clean Git history.
Download, build and install Corrade and Magnum
Magnum libraries support both separate compilation/installation and CMake subprojects. If you are lucky, you may already have Magnum packages ready for your platform and you can skip the rest of this section:
- Vcpkg packages on Windows
- ArchLinux packages
- Packages for Debian, Ubuntu and derivatives
- Gentoo Linux ebuilds
- Homebrew formulas for macOS
If you don't, don't worry, let's use the subproject approach instead. Adding the dependencies means just cloning them into your project tree:
cd /path/to/the/extracted/bootstrap/project
git clone git://github.com/mosra/corrade.git
git clone git://github.com/mosra/magnum.git
Then open the CMakeLists.txt
file in the root of bootstrap project and add these two new subdirectories using add_subdirectory()
so the file looks like this:
cmake_minimum_required(VERSION 2.8.12) project(MyApplication) set(CMAKE_MODULE_PATH ${CMAKE_MODULE_PATH} "${PROJECT_SOURCE_DIR}/modules/") add_subdirectory(corrade) add_subdirectory(magnum) add_subdirectory(src)
Review project structure
The base project consists of just six files in two subfolders. Magnum uses the CMake build system, you can read more about it in Usage with CMake.
modules/FindCorrade.cmake modules/FindMagnum.cmake modules/FindSDL2.cmake src/MyApplication.cpp src/CMakeLists.txt CMakeLists.txt
In root there is the project-wide CMakeLists.txt
, which you have seen above. It just sets up project name, specifies module directory and delegates everything important to CMakeLists.txt
in the src/
subdirectory.
The modules/
directory contains CMake modules for finding the needed dependencies. Unlike modules for finding e.g. OpenGL, which are part of standard CMake installation, these aren't part of it and thus must be distributed with the project. These files are just verbatim copied from Magnum repository.
The src/
directory contains the actual project. To keep things simple, the project consists of just a single MyApplication.cpp
file with the most minimal code possible:
#include <Magnum/GL/DefaultFramebuffer.h> #include <Magnum/Platform/Sdl2Application.h> using namespace Magnum; class MyApplication: public Platform::Application { public: explicit MyApplication(const Arguments& arguments); private: void drawEvent() override; }; MyApplication::MyApplication(const Arguments& arguments): Platform::Application{arguments} { // TODO: Add your initialization code here } void MyApplication::drawEvent() { GL::defaultFramebuffer.clear(GL::FramebufferClear::Color); // TODO: Add your drawing code here swapBuffers(); } MAGNUM_APPLICATION_MAIN(MyApplication)
The application essentially does nothing, just clears the screen framebuffer to default (dark gray) color and then does buffer swap to actually display it on the screen. The src/CMakeLists.txt
file finds Magnum, creates the executable and links it to all needed libraries:
find_package(Magnum REQUIRED Sdl2Application) set_directory_properties(PROPERTIES CORRADE_USE_PEDANTIC_FLAGS ON) add_executable(MyApplication MyApplication.cpp) target_link_libraries(MyApplication PRIVATE Magnum::Magnum Magnum::Application)
In the following tutorials the code will be explained more thoroughly.
Build it and run
Linux, macOS and other Unix-based OSes
In Linux (and other Unix-based OSs) you can build the application along with the subprojects using the following three commands: create out-of-source build directory, run cmake
, enable SDL2 application in the Magnum subproject and then build everything. The compiled application binary will then appear in src/
subdirectory of the build dir:
mkdir -p build && cd build cmake .. -DWITH_SDL2APPLICATION=ON cmake --build . ./src/MyApplication
Windows
On Windows you can use either MSVC 2015+ or MinGW-w64. Prebuilt SDL2 binaries can be downloaded at https:/CMAKE_PREFIX_PATH
so CMake is able to find them. For running the executable properly, Windows also need to have all dependency DLLs copied along it. That can be done by setting CMAKE_RUNTIME_OUTPUT_DIRECTORY
. It's then up to you whether you will use a command line, Visual Studio or for example QtCreator. With Visual Studio the most straightforward way to generate the project file is via the command line:
mkdir build && cd build cmake .. ^ -DWITH_SDL2APPLICATION=ON ^ -DCMAKE_PREFIX_PATH="C:/Users/you/where/you/extracted/SDL2-2.0.5" ^ -DCMAKE_RUNTIME_OUTPUT_DIRECTORY="bin"
You can also use CMake GUI. Then open the MyApplication.sln
project file generated by CMake in the build/
directory.
With QtCreator just open project's root CMakeLists.txt
file. It then asks you where to create build directory, allows you to specify initial CMake parameters (-DWITH_SDL2APPLICATION=ON
and others) and then you can just press Configure and everything is ready to be built.
Running the application
If everything went well and the application starts, you will see a blank window like this:
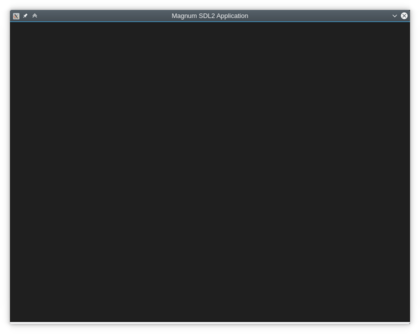
Now you can try to change something in the code. Without going too deep into the concepts of graphics programming, we can change the clear color to something else and also print basic information about the GPU the engine is running on. First include the needed headers:
#include <Magnum/GL/Context.h> #include <Magnum/GL/Renderer.h> #include <Magnum/GL/Version.h> #include <Magnum/Math/Color.h>
And in the constructor (which is currently empty) change the clear color and print something to debug output:
MyApplication::MyApplication(const Arguments& arguments): Platform::Application{arguments} { using namespace Magnum::Math::Literals; GL::Renderer::setClearColor(0xa5c9ea_rgbf); Debug{} << "Hello! This application is running on" << GL::Context::current().version() << "using" << GL::Context::current().rendererString(); }
After rebuilding and starting the application, the clear color changes to blueish one and something like this would be printed to the console:
$ ./MyApplication [...] Hello! This application is running on OpenGL 4.5 using GeForce GT 740M
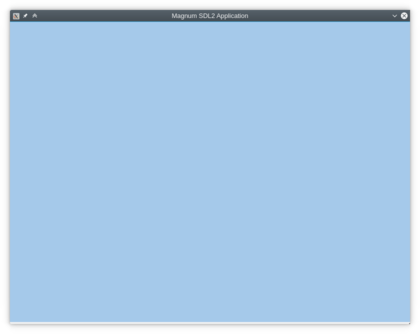
Follow tutorials and learn the principles
Now that you have your first application up and running, the best way to continue is to render your first triangle in a step-by-step tutorial. Then you can dig deeper and try other examples, read about fundamental principles in the documentation and start experimenting on your own!
Additional information
- Downloading and building — Guide how to download and build Magnum on different platforms.
- Downloading and building plugins — Guide how to download and build plugins for the Magnum engine.
- Downloading and building integration libraries — Guide how to download and build integration libraries for the Magnum engine.
- Downloading and building extras — Guide how to download and build extras for Magnum engine.
- Downloading and building examples — Guide how to download and build examples for the Magnum engine.
- Usage with CMake — Guide how to find and use Magnum with CMake build system.
- Plugin usage with CMake — Guide how to find and use static Magnum plugins with CMake build system.
- Integration library usage with CMake — Guide how to find and use Magnum integration libraries with CMake build system.
- Extras library usage with CMake — Guide how to find and use Magnum Extras with CMake build system.