template<UnsignedInt dimensions>
Vector class
Vector shader.
Contents
Renders vector art in plain grayscale form. See also DistanceFieldVector for more advanced effects. For rendering unchanged texture you can use the Flat shader. You need to provide Position and TextureCoordinates attributes in your triangle mesh and call at least setTransformationProjectionMatrix(), setColor() and bindVectorTexture().
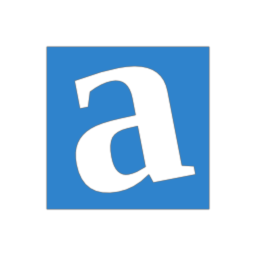
Example usage
Common mesh setup:
struct Vertex { Vector2 position; Vector2 textureCoordinates; }; Vertex data[60]{ // ... }; GL::Buffer vertices; vertices.setData(data, GL::BufferUsage::StaticDraw); GL::Mesh mesh; mesh.addVertexBuffer(vertices, 0, Shaders::Vector2D::Position{}, Shaders::Vector2D::TextureCoordinates{});
Common rendering setup:
Matrix3 transformationMatrix, projectionMatrix; GL::Texture2D texture; Shaders::Vector2D shader; shader.setColor(0x2f83cc_rgbf) .bindVectorTexture(texture) .setTransformationProjectionMatrix(projectionMatrix*transformationMatrix); mesh.draw(shader);
Base classes
-
template<UnsignedInt dimensions>class AbstractVector
- Base for vector shaders.
Constructors, destructors, conversion operators
Public functions
- auto setTransformationProjectionMatrix(const MatrixTypeFor<dimensions, Float>& matrix) -> Vector&
- Set transformation and projection matrix.
- auto setBackgroundColor(const Color4& color) -> Vector&
- Set background color.
- auto setColor(const Color4& color) -> Vector&
- Set fill color.
Function documentation
template<UnsignedInt dimensions>
Magnum:: Shaders:: Vector<dimensions>:: Vector(NoCreateT) explicit noexcept
Construct without creating the underlying OpenGL object.
The constructed instance is equivalent to moved-from state. Useful in cases where you will overwrite the instance later anyway. Move another object over it to make it useful.
This function can be safely used for constructing (and later destructing) objects even without any OpenGL context being active.
template<UnsignedInt dimensions>
Vector& Magnum:: Shaders:: Vector<dimensions>:: setTransformationProjectionMatrix(const MatrixTypeFor<dimensions, Float>& matrix)
Set transformation and projection matrix.
Returns | Reference to self (for method chaining) |
---|
template<UnsignedInt dimensions>
Vector& Magnum:: Shaders:: Vector<dimensions>:: setBackgroundColor(const Color4& color)
Set background color.
Returns | Reference to self (for method chaining) |
---|
Default is transparent black.
template<UnsignedInt dimensions>
Vector& Magnum:: Shaders:: Vector<dimensions>:: setColor(const Color4& color)
Set fill color.
Returns | Reference to self (for method chaining) |
---|