class
PhongPhong shader.
Contents
Uses ambient, diffuse and specular color or texture. For colored mesh you need to provide Position and Normal attributes in your triangle mesh and call at least setTransformationMatrix(), setNormalMatrix(), setProjectionMatrix(), setDiffuseColor() and setLightPosition().
If you want to use textures, you need to provide also TextureCoordinates attribute. Pass appropriate Flags to constructor and then at render time don't forget to also call appropriate subset of bindAmbientTexture(), bindDiffuseTexture() and bindSpecularTexture() (or the combined bindTextures()). The texture is multipled by the color, which is by default set to fully opaque white for enabled textures.
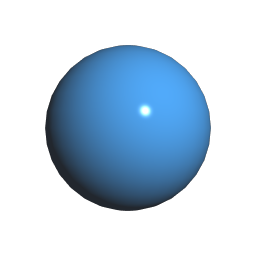
Example usage
Colored mesh
Common mesh setup:
struct Vertex { Vector3 position; Vector3 normal; }; Vertex data[60]{ // ... }; GL::Buffer vertices; vertices.setData(data, GL::BufferUsage::StaticDraw); GL::Mesh mesh; mesh.addVertexBuffer(vertices, 0, Shaders::Phong::Position{}, Shaders::Phong::Normal{});
Common rendering setup:
Matrix4 transformationMatrix = Matrix4::translation(Vector3::zAxis(-5.0f)); Matrix4 projectionMatrix = Matrix4::perspectiveProjection(35.0_degf, 1.0f, 0.001f, 100.0f); Shaders::Phong shader; shader.setDiffuseColor(0x2f83cc_rgbf) .setShininess(200.0f) .setLightPosition({5.0f, 5.0f, 7.0f}) .setTransformationMatrix(transformationMatrix) .setNormalMatrix(transformationMatrix.rotation()) .setProjectionMatrix(projectionMatrix); mesh.draw(shader);
Diffuse and specular texture
Common mesh setup:
struct Vertex { Vector3 position; Vector3 normal; Vector2 textureCoordinates; }; Vertex data[60]{ // ... }; GL::Buffer vertices; vertices.setData(data, GL::BufferUsage::StaticDraw); GL::Mesh mesh; mesh.addVertexBuffer(vertices, 0, Shaders::Phong::Position{}, Shaders::Phong::Normal{}, Shaders::Phong::TextureCoordinates{});
Common rendering setup:
Matrix4 transformationMatrix, projectionMatrix; GL::Texture2D diffuseTexture, specularTexture; Shaders::Phong shader{Shaders::Phong::Flag::DiffuseTexture| Shaders::Phong::Flag::SpecularTexture}; shader.bindTextures(nullptr, &diffuseTexture, &specularTexture) .setLightPosition({5.0f, 5.0f, 7.0f}) .setTransformationMatrix(transformationMatrix) .setNormalMatrix(transformationMatrix.rotation()) .setProjectionMatrix(projectionMatrix); mesh.draw(shader);
Alpha-masked drawing
For general alpha-masked drawing you need to provide ambient texture with alpha channel and set alpha channel of diffuse/specular color to 0.0f
so only ambient alpha will be taken into account. If you have diffuse texture combined with the alpha mask, you can use that texture for both ambient and diffuse part and then separate the alpha like this:
Shaders::Phong shader{Shaders::Phong::Flag::AmbientTexture| Shaders::Phong::Flag::DiffuseTexture}; shader.bindTextures(&ambientAlphaTexture, &diffuseAlphaTexture, nullptr) .setAmbientColor(0x000000ff_rgbf) .setDiffuseColor(Color4{diffuseRgb, 0.0f}) .setSpecularColor(Color4{specularRgb, 0.0f});
Base classes
- class Magnum::GL::AbstractShaderProgram
- Base for shader program implementations.
Public types
- enum class Flag: UnsignedByte { AmbientTexture = 1 << 0, DiffuseTexture = 1 << 1, SpecularTexture = 1 << 2 }
- Flag.
-
using Position = Generic3D::
Position - Vertex position.
-
using Normal = Generic3D::
Normal - Normal direction.
-
using TextureCoordinates = Generic3D::
TextureCoordinates - 2D texture coordinates
-
using Flags = Containers::
EnumSet <Flag> - Flags.
Constructors, destructors, conversion operators
Public functions
- auto flags() const -> Flags
- Flags.
- auto setAmbientColor(const Color4& color) -> Phong&
- Set ambient color.
-
auto bindAmbientTexture(GL::
Texture2D & texture) -> Phong& - Bind ambient texture.
-
auto setAmbientTexture(GL::
Texture2D & texture) -> Phong& deprecated - Bind ambient texture.
- auto setDiffuseColor(const Color4& color) -> Phong&
- Set diffuse color.
-
auto bindDiffuseTexture(GL::
Texture2D & texture) -> Phong& - Bind diffuse texture.
-
auto setDiffuseTexture(GL::
Texture2D & texture) -> Phong& deprecated - Bind diffuse texture.
- auto setSpecularColor(const Color4& color) -> Phong&
- Set specular color.
-
auto bindSpecularTexture(GL::
Texture2D & texture) -> Phong& - Bind specular texture.
-
auto setSpecularTexture(GL::
Texture2D & texture) -> Phong& deprecated - Bind specular texture.
-
auto bindTextures(GL::
Texture2D * ambient, GL::Texture2D * diffuse, GL::Texture2D * specular) -> Phong& - Bind textures.
-
auto setTextures(GL::
Texture2D * ambient, GL::Texture2D * diffuse, GL::Texture2D * specular) -> Phong& deprecated - Bind textures.
- auto setShininess(Float shininess) -> Phong&
- Set shininess.
- auto setTransformationMatrix(const Matrix4& matrix) -> Phong&
- Set transformation matrix.
- auto setNormalMatrix(const Matrix3x3& matrix) -> Phong&
- Set normal matrix.
- auto setProjectionMatrix(const Matrix4& matrix) -> Phong&
- Set projection matrix.
- auto setLightPosition(const Vector3& light) -> Phong&
- Set light position.
- auto setLightColor(const Color4& color) -> Phong&
- Set light color.
Enum documentation
enum class Magnum::Shaders::Phong::Flag: UnsignedByte
Flag.
Enumerators | |
---|---|
AmbientTexture |
The shader uses ambient texture instead of color |
DiffuseTexture |
The shader uses diffuse texture instead of color |
SpecularTexture |
The shader uses specular texture instead of color |
Typedef documentation
typedef Generic3D::Position Magnum::Shaders::Phong::Position
Vertex position.
typedef Generic3D::Normal Magnum::Shaders::Phong::Normal
Normal direction.
typedef Generic3D::TextureCoordinates Magnum::Shaders::Phong::TextureCoordinates
2D texture coordinates
Generic attribute, Vector2, used only if at least one of Flag::
typedef Containers::EnumSet <Flag> Magnum::Shaders::Phong::Flags
Flags.
Function documentation
Magnum::Shaders::Phong:: Phong(NoCreateT) explicit noexcept
Construct without creating the underlying OpenGL object.
The constructed instance is equivalent to moved-from state. Useful in cases where you will overwrite the instance later anyway. Move another object over it to make it useful.
This function can be safely used for constructing (and later destructing) objects even without any OpenGL context being active.
Phong& Magnum::Shaders::Phong:: setAmbientColor(const Color4& color)
Set ambient color.
Returns | Reference to self (for method chaining) |
---|
If Flag::0xffffffff_rgbaf
and the color will be multiplied with ambient texture, otherwise default value is 0x000000ff_rgbaf
.
Phong& Magnum::Shaders::Phong:: bindAmbientTexture(GL::Texture2D & texture)
Bind ambient texture.
Returns | Reference to self (for method chaining) |
---|
Has effect only if Flag::
Phong& Magnum::Shaders::Phong:: setAmbientTexture(GL::Texture2D & texture)
Bind ambient texture.
Phong& Magnum::Shaders::Phong:: setDiffuseColor(const Color4& color)
Set diffuse color.
Returns | Reference to self (for method chaining) |
---|
If Flag::0xffffffff_rgbaf
and the color will be multiplied with diffuse texture.
Phong& Magnum::Shaders::Phong:: bindDiffuseTexture(GL::Texture2D & texture)
Bind diffuse texture.
Returns | Reference to self (for method chaining) |
---|
Has effect only if Flag::
Phong& Magnum::Shaders::Phong:: setDiffuseTexture(GL::Texture2D & texture)
Bind diffuse texture.
Phong& Magnum::Shaders::Phong:: setSpecularColor(const Color4& color)
Set specular color.
Returns | Reference to self (for method chaining) |
---|
Default value is 0xffffffff_rgbaf
. Color will be multiplied with specular texture if Flag::0x000000_rgbf
.
Phong& Magnum::Shaders::Phong:: bindSpecularTexture(GL::Texture2D & texture)
Bind specular texture.
Returns | Reference to self (for method chaining) |
---|
Has effect only if Flag::
Phong& Magnum::Shaders::Phong:: setSpecularTexture(GL::Texture2D & texture)
Bind specular texture.
Phong& Magnum::Shaders::Phong:: bindTextures(GL::Texture2D * ambient,
GL::Texture2D * diffuse,
GL::Texture2D * specular)
Bind textures.
Returns | Reference to self (for method chaining) |
---|
A particular texture has effect only if particular texture flag from Flag is set, you can use nullptr
for the rest. More efficient than setting each texture separately.
Phong& Magnum::Shaders::Phong:: setTextures(GL::Texture2D * ambient,
GL::Texture2D * diffuse,
GL::Texture2D * specular)
Bind textures.
Phong& Magnum::Shaders::Phong:: setShininess(Float shininess)
Set shininess.
Returns | Reference to self (for method chaining) |
---|
The larger value, the harder surface (smaller specular highlight). If not set, default value is 80.0f
.
Phong& Magnum::Shaders::Phong:: setTransformationMatrix(const Matrix4& matrix)
Set transformation matrix.
Returns | Reference to self (for method chaining) |
---|
Phong& Magnum::Shaders::Phong:: setNormalMatrix(const Matrix3x3& matrix)
Set normal matrix.
Returns | Reference to self (for method chaining) |
---|
The matrix doesn't need to be normalized, as the renormalization must be done in the shader anyway.
Phong& Magnum::Shaders::Phong:: setProjectionMatrix(const Matrix4& matrix)
Set projection matrix.
Returns | Reference to self (for method chaining) |
---|
Phong& Magnum::Shaders::Phong:: setLightPosition(const Vector3& light)
Set light position.
Returns | Reference to self (for method chaining) |
---|
Phong& Magnum::Shaders::Phong:: setLightColor(const Color4& color)
Set light color.
Returns | Reference to self (for method chaining) |
---|
If not set, default value is 0xffffffff_rgbaf
.