template<UnsignedInt dimensions>
VertexColor class
Vertex color shader.
Contents
Draws vertex-colored mesh. You need to provide Position and Color attributes in your triangle mesh and call at least setTransformationProjectionMatrix().
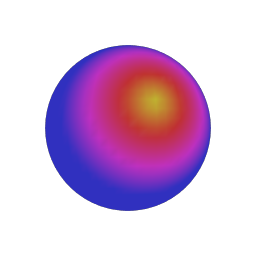
Example usage
Common mesh setup. Note the explicit specification of components for the color attribute — the shader accepts four-component color attribute but, similarly to all other attributes, it's possible to supply also three-component colors if alpha is not important.
struct Vertex { Vector3 position; Color3 color; }; Vertex data[60]{ // ... }; GL::Buffer vertices; vertices.setData(data, GL::BufferUsage::StaticDraw); GL::Mesh mesh; mesh.addVertexBuffer(vertices, 0, Shaders::VertexColor3D::Position{}, Shaders::VertexColor3D::Color{Shaders::VertexColor3D::Color::Components::Three});
Common rendering setup:
Matrix4 transformationMatrix = Matrix4::translation(Vector3::zAxis(-5.0f)); Matrix4 projectionMatrix = Matrix4::perspectiveProjection(35.0_degf, 1.0f, 0.001f, 100.0f); Shaders::VertexColor3D shader; shader.setTransformationProjectionMatrix(projectionMatrix*transformationMatrix); mesh.draw(shader);
Base classes
- class Magnum::GL::AbstractShaderProgram
- Base for shader program implementations.
Public types
Constructors, destructors, conversion operators
- VertexColor(NoCreateT) explicit noexcept
- Construct without creating the underlying OpenGL object.
Public functions
- auto setTransformationProjectionMatrix(const MatrixTypeFor<dimensions, Float>& matrix) -> VertexColor<dimensions>&
- Set transformation and projection matrix.
Typedef documentation
template<UnsignedInt dimensions>
typedef Generic<dimensions>::Position Magnum:: Shaders:: VertexColor<dimensions>:: Position
Vertex position.
Generic attribute, Vector2 in 2D, Vector3 in 3D.
template<UnsignedInt dimensions>
typedef Generic<dimensions>::Color Magnum:: Shaders:: VertexColor<dimensions>:: Color
Vertex color.
Generic attribute, Color4, however defaults to Color3 if MAGNUM_
Function documentation
template<UnsignedInt dimensions>
Magnum:: Shaders:: VertexColor<dimensions>:: VertexColor(NoCreateT) explicit noexcept
Construct without creating the underlying OpenGL object.
The constructed instance is equivalent to moved-from state. Useful in cases where you will overwrite the instance later anyway. Move another object over it to make it useful.
This function can be safely used for constructing (and later destructing) objects even without any OpenGL context being active.
template<UnsignedInt dimensions>
VertexColor<dimensions>& Magnum:: Shaders:: VertexColor<dimensions>:: setTransformationProjectionMatrix(const MatrixTypeFor<dimensions, Float>& matrix)
Set transformation and projection matrix.
Returns | Reference to self (for method chaining) |
---|
Default is identity matrix.