namespace
PrimitivesPrimitive library.
Basic primitives for testing purposes.
This library is built if WITH_PRIMITIVES
is enabled when building Magnum. To use this library with CMake, you need to request the Primitives
component of the Magnum
package and link to the Magnum::Primitives
target:
find_package(Magnum REQUIRED Primitives) # ... target_link_libraries(your-app Magnum::Primitives)
See Downloading and building and Usage with CMake for more information.
Classes
- struct Capsule2D deprecated
- 2D capsule
- struct Capsule3D deprecated
- 3D capsule
- struct Circle deprecated
- 2D circle primitive
- struct Crosshair2D deprecated
- 2D crosshair
- struct Crosshair3D deprecated
- 3D crosshair
- struct Cube deprecated
- 3D cube
- struct Cylinder deprecated
- 3D cylinder
- struct Icosphere deprecated
- 3D icosphere
- struct Line2D deprecated
- 2D line
- struct Line3D deprecated
- 3D line
- struct Plane deprecated
- 3D plane
- struct Square deprecated
- 2D square
- struct UVSphere deprecated
- 3D UV sphere
Enums
- enum class CapsuleTextureCoords: UnsignedByte { DontGenerate, Generate }
- Whether to generate capsule texture coordinates.
- enum class ConeFlag { GenerateTextureCoords = 1 << 0, CapEnd = 1 << 1 }
- Cone flag.
- enum class CylinderFlag { GenerateTextureCoords = 1 << 0, CapEnds = 1 << 1 }
- Cylinder flag.
- enum class GridFlag: UnsignedByte { GenerateTextureCoords = 1 << 0, GenerateNormals = 1 << 1 }
- Grid flag.
- enum class PlaneTextureCoords: UnsignedByte { DontGenerate, Generate }
- Whether to generate plane texture coordinates.
- enum class SquareTextureCoords: UnsignedByte { DontGenerate, Generate }
- Whether to generate square texture coordinates.
- enum class UVSphereTextureCoords: UnsignedByte { DontGenerate, Generate }
- Whether to generate UV sphere texture coordinates.
Typedefs
-
using ConeFlags = Containers::
EnumSet<ConeFlag> - Cone flags.
-
using CylinderFlags = Containers::
EnumSet<CylinderFlag> - Cylinder flags.
-
using GridFlags = Containers::
EnumSet<GridFlag> - Grid flags.
Functions
-
auto axis2D() -> Trade::
MeshData2D - 2D axis
-
auto axis3D() -> Trade::
MeshData3D - 3D axis
-
auto capsule2DWireframe(UnsignedInt hemisphereRings,
UnsignedInt cylinderRings,
Float halfLength) -> Trade::
MeshData2D - Wireframe 2D capsule.
-
auto capsule3DSolid(UnsignedInt hemisphereRings,
UnsignedInt cylinderRings,
UnsignedInt segments,
Float halfLength,
CapsuleTextureCoords textureCoords = CapsuleTextureCoords::
DontGenerate) -> Trade:: MeshData3D - Solid 3D capsule.
-
auto capsule3DWireframe(UnsignedInt hemisphereRings,
UnsignedInt cylinderRings,
UnsignedInt segments,
Float halfLength) -> Trade::
MeshData3D - Wireframe 3D capsule.
-
auto circle2DSolid(UnsignedInt segments) -> Trade::
MeshData2D - Solid 2D circle.
-
auto circle2DWireframe(UnsignedInt segments) -> Trade::
MeshData2D - Wireframe 2D circle.
-
auto circle3DSolid(UnsignedInt segments) -> Trade::
MeshData3D - Solid 3D circle.
-
auto circle3DWireframe(UnsignedInt segments) -> Trade::
MeshData3D - Wireframe 3D circle.
-
auto coneSolid(UnsignedInt rings,
UnsignedInt segments,
Float halfLength,
ConeFlags flags = {}) -> Trade::
MeshData3D - Solid 3D cone.
-
auto coneWireframe(UnsignedInt segments,
Float halfLength) -> Trade::
MeshData3D - Wireframe 3D cone.
-
auto crosshair2D() -> Trade::
MeshData2D - 2D crosshair
-
auto crosshair3D() -> Trade::
MeshData3D - 3D crosshair
-
auto cubeSolid() -> Trade::
MeshData3D - Solid 3D cube.
-
auto cubeSolidStrip() -> Trade::
MeshData3D - Solid 3D cube as a single strip.
-
auto cubeWireframe() -> Trade::
MeshData3D - Wireframe 3D cube.
-
auto cylinderSolid(UnsignedInt rings,
UnsignedInt segments,
Float halfLength,
CylinderFlags flags = {}) -> Trade::
MeshData3D - Solid 3D cylinder.
-
auto cylinderWireframe(UnsignedInt rings,
UnsignedInt segments,
Float halfLength) -> Trade::
MeshData3D - Wireframe 3D cylinder.
-
auto grid3DSolid(const Vector2i& subdivisions,
GridFlags flags = GridFlag::
GenerateNormals) -> Trade:: MeshData3D - 3D solid grid
-
auto grid3DWireframe(const Vector2i& subdivisions) -> Trade::
MeshData3D - 3D wireframe grid
-
auto icosphereSolid(UnsignedInt subdivisions) -> Trade::
MeshData3D - Solid 3D icosphere.
-
auto line2D() -> Trade::
MeshData2D - 2D line
-
auto line3D() -> Trade::
MeshData3D - 3D line
-
auto planeSolid(PlaneTextureCoords textureCoords = PlaneTextureCoords::
DontGenerate) -> Trade:: MeshData3D - Solid 3D plane.
-
auto planeWireframe() -> Trade::
MeshData3D - Wireframe 3D plane.
-
auto squareSolid(SquareTextureCoords textureCoords = SquareTextureCoords::
DontGenerate) -> Trade:: MeshData2D - Solid 2D square.
-
auto squareWireframe() -> Trade::
MeshData2D - Wireframe 2D square.
-
auto uvSphereSolid(UnsignedInt rings,
UnsignedInt segments,
UVSphereTextureCoords textureCoords = UVSphereTextureCoords::
DontGenerate) -> Trade:: MeshData3D - Solid 3D UV sphere.
-
auto uvSphereWireframe(UnsignedInt rings,
UnsignedInt segments) -> Trade::
MeshData3D - Wireframe 3D UV sphere.
Enum documentation
enum class Magnum:: Primitives:: CapsuleTextureCoords: UnsignedByte
Whether to generate capsule texture coordinates.
Enumerators | |
---|---|
DontGenerate |
Don't generate texture coordinates |
Generate |
Generate texture coordinates |
enum class Magnum:: Primitives:: ConeFlag
Cone flag.
Enumerators | |
---|---|
GenerateTextureCoords |
Generate texture coordinates |
CapEnd |
Cap end |
enum class Magnum:: Primitives:: CylinderFlag
Cylinder flag.
Enumerators | |
---|---|
GenerateTextureCoords |
Generate texture coordinates |
CapEnds |
Cap ends |
enum class Magnum:: Primitives:: GridFlag: UnsignedByte
Grid flag.
Enumerators | |
---|---|
GenerateTextureCoords |
Generate texture coordinates with origin in bottom left corner. |
GenerateNormals |
Generate normals inn positive Z direction. Disable if you'd be generating your own normals anyway (for example based on a heightmap). |
enum class Magnum:: Primitives:: PlaneTextureCoords: UnsignedByte
Whether to generate plane texture coordinates.
Enumerators | |
---|---|
DontGenerate |
Don't generate texture coordinates |
Generate |
Generate texture coordinates with origin in bottom left corner. |
enum class Magnum:: Primitives:: SquareTextureCoords: UnsignedByte
Whether to generate square texture coordinates.
Enumerators | |
---|---|
DontGenerate |
Don't generate texture coordinates |
Generate |
Generate texture coordinates with origin in bottom left corner. |
enum class Magnum:: Primitives:: UVSphereTextureCoords: UnsignedByte
Whether to generate UV sphere texture coordinates.
Enumerators | |
---|---|
DontGenerate |
Don't generate texture coordinates |
Generate |
Generate texture coordinates |
Typedef documentation
typedef Containers:: EnumSet<ConeFlag> Magnum:: Primitives:: ConeFlags
Cone flags.
typedef Containers:: EnumSet<CylinderFlag> Magnum:: Primitives:: CylinderFlags
Cylinder flags.
typedef Containers:: EnumSet<GridFlag> Magnum:: Primitives:: GridFlags
Grid flags.
Function documentation
Trade:: MeshData2D Magnum:: Primitives:: axis2D()
2D axis
Two color-coded arrows for visualizing orientation (XY is RG). Indexed MeshPrimitive::
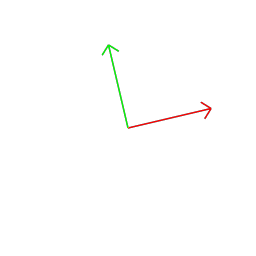
Trade:: MeshData3D Magnum:: Primitives:: axis3D()
3D axis
Three color-coded arrows for visualizing orientation (XYZ is RGB). Indexed MeshPrimitive::
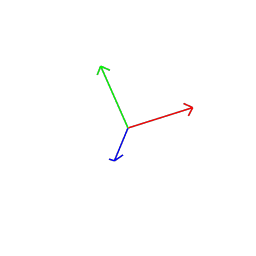
Trade:: MeshData2D Magnum:: Primitives:: capsule2DWireframe(UnsignedInt hemisphereRings,
UnsignedInt cylinderRings,
Float halfLength)
Wireframe 2D capsule.
Parameters | |
---|---|
hemisphereRings | Number of (line) rings for each hemisphere. Must be larger or equal to 1 . |
cylinderRings | Number of (line) rings for cylinder. Must be larger or equal to 1 . |
halfLength | Half the length of cylinder part |
Cylinder of radius 1.0f
along Y axis with hemispheres instead of caps. Indexed MeshPrimitive::
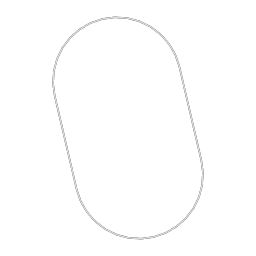
Trade:: MeshData3D Magnum:: Primitives:: capsule3DSolid(UnsignedInt hemisphereRings,
UnsignedInt cylinderRings,
UnsignedInt segments,
Float halfLength,
CapsuleTextureCoords textureCoords = CapsuleTextureCoords:: DontGenerate)
Solid 3D capsule.
Parameters | |
---|---|
hemisphereRings | Number of (face) rings for each hemisphere. Must be larger or equal to 1 . |
cylinderRings | Number of (face) rings for cylinder. Must be larger or equal to 1 . |
segments | Number of (face) segments. Must be larger or equal to 3 . |
halfLength | Half the length of cylinder part |
textureCoords | Whether to generate texture coordinates |
Cylinder of radius 1.0f
along Y axis with hemispheres instead of caps. Indexed MeshPrimitive::
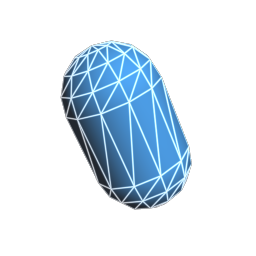
The capsule is by default created with radius set to . In order to get radius , length and preserve correct normals, set halfLength
to and then scale all Trade::
Trade:: MeshData3D Magnum:: Primitives:: capsule3DWireframe(UnsignedInt hemisphereRings,
UnsignedInt cylinderRings,
UnsignedInt segments,
Float halfLength)
Wireframe 3D capsule.
Parameters | |
---|---|
hemisphereRings | Number of (line) rings for each hemisphere. Must be larger or equal to 1 . |
cylinderRings | Number of (line) rings for cylinder. Must be larger or equal to 1 . |
segments | Number of line segments. Must be larger or equal to 4 and multiple of 4 . |
halfLength | Half the length of cylinder part |
Cylinder of radius 1.0f
along Y axis with hemispheres instead of caps. Indexed MeshPrimitive::
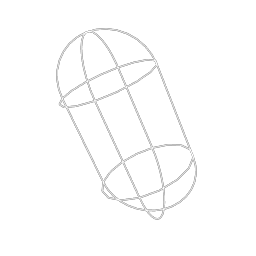
Trade:: MeshData2D Magnum:: Primitives:: circle2DSolid(UnsignedInt segments)
Solid 2D circle.
Parameters | |
---|---|
segments | Number of segments. Must be greater or equal to 3 . |
Circle with radius 1.0f
. Non-indexed MeshPrimitive::
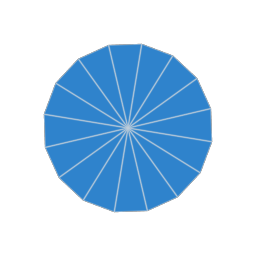
Trade:: MeshData2D Magnum:: Primitives:: circle2DWireframe(UnsignedInt segments)
Wireframe 2D circle.
Parameters | |
---|---|
segments | Number of segments. Must be greater or equal to 3 . |
Circle with radius 1.0f
. Non-indexed MeshPrimitive::
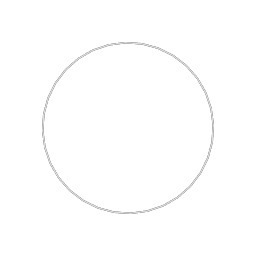
Trade:: MeshData3D Magnum:: Primitives:: circle3DSolid(UnsignedInt segments)
Solid 3D circle.
Parameters | |
---|---|
segments | Number of segments. Must be greater or equal to 3 . |
Circle on the XY plane with radius 1.0f
. Non-indexed MeshPrimitive::
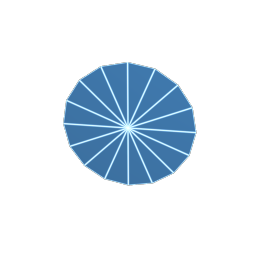
Trade:: MeshData3D Magnum:: Primitives:: circle3DWireframe(UnsignedInt segments)
Wireframe 3D circle.
Parameters | |
---|---|
segments | Number of segments. Must be greater or equal to 3 . |
Circle on the XY plane with radius 1.0f
. Non-indexed MeshPrimitive::
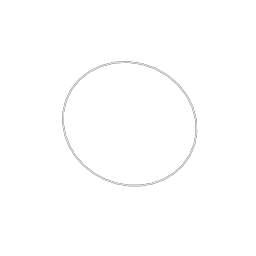
Trade:: MeshData3D Magnum:: Primitives:: coneSolid(UnsignedInt rings,
UnsignedInt segments,
Float halfLength,
ConeFlags flags = {})
Solid 3D cone.
Parameters | |
---|---|
rings | Number of (face) rings. Must be larger or equal to 1 . |
segments | Number of (face) segments. Must be larger or equal to 3 . |
halfLength | Half the cone length |
flags | Flags |
Cone along Y axis of radius 1.0f
. Indexed MeshPrimitive::segments*2
vertices instead of just one.
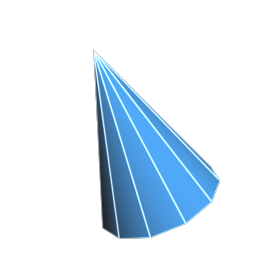
The cone is by default created with radius set to . In order to get radius , length and preserve correct normals, set halfLength
to and then scale all Trade::
Trade:: MeshData3D Magnum:: Primitives:: coneWireframe(UnsignedInt segments,
Float halfLength)
Wireframe 3D cone.
Parameters | |
---|---|
segments | Number of (line) segments. Must be larger or equal to 4 and multiple of 4 . |
halfLength | Half the cone length |
Cone along Y axis of radius 1.0f
. Indexed MeshPrimitive::
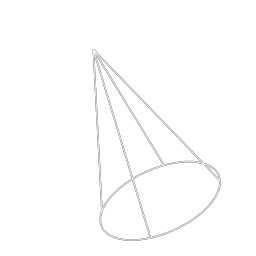
Trade:: MeshData2D Magnum:: Primitives:: crosshair2D()
2D crosshair
2x2 crosshair (two crossed lines), non-indexed MeshPrimitive::
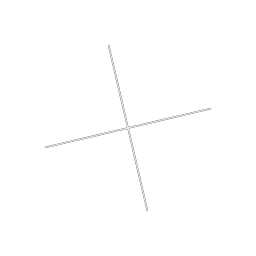
Trade:: MeshData3D Magnum:: Primitives:: crosshair3D()
3D crosshair
2x2x2 crosshair (three crossed lines), non-indexed MeshPrimitive::
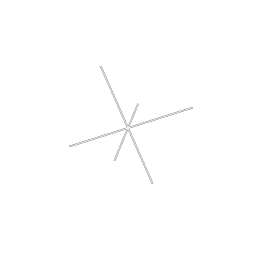
Trade:: MeshData3D Magnum:: Primitives:: cubeSolid()
Solid 3D cube.
Indexed MeshPrimitive::
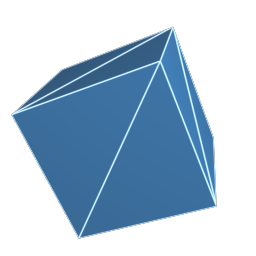
Trade:: MeshData3D Magnum:: Primitives:: cubeSolidStrip()
Solid 3D cube as a single strip.
Non-indexed MeshPrimitive::
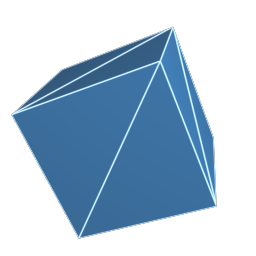
Trade:: MeshData3D Magnum:: Primitives:: cubeWireframe()
Wireframe 3D cube.
Indexed MeshPrimitive::
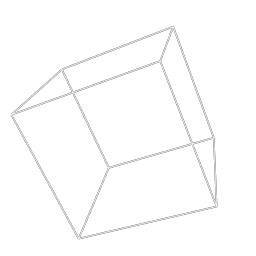
Trade:: MeshData3D Magnum:: Primitives:: cylinderSolid(UnsignedInt rings,
UnsignedInt segments,
Float halfLength,
CylinderFlags flags = {})
Solid 3D cylinder.
Parameters | |
---|---|
rings | Number of (face) rings. Must be larger or equal to 1 . |
segments | Number of (face) segments. Must be larger or equal to 3 . |
halfLength | Half the cylinder length |
flags | Flags |
Cylinder along Y axis of radius 1.0f
. Indexed MeshPrimitive::
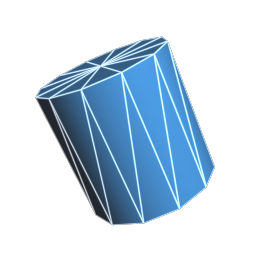
The cylinder is by default created with radius set to . In order to get radius , length and preserve correct normals, set halfLength
to and then scale all Trade::
Trade:: MeshData3D Magnum:: Primitives:: cylinderWireframe(UnsignedInt rings,
UnsignedInt segments,
Float halfLength)
Wireframe 3D cylinder.
Parameters | |
---|---|
rings | Number of (line) rings. Must be larger or equal to 1 . |
segments | Number of (line) segments. Must be larger or equal to 4 and multiple of 4 . |
halfLength | Half the cylinder length |
Cylinder along Y axis of radius 1.0f
. Indexed MeshPrimitive::
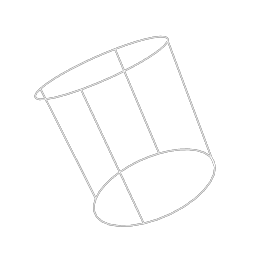
Trade:: MeshData3D Magnum:: Primitives:: grid3DSolid(const Vector2i& subdivisions,
GridFlags flags = GridFlag:: GenerateNormals)
3D solid grid
2x2 grid. Indexed MeshPrimitive::
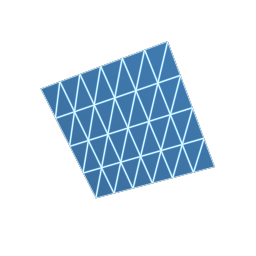
The subdivisions
parameter describes how many times the plane gets cut in each direction. Specifying {0, 0}
will make the result an (indexed) equivalent to planeSolid(); {5, 3}
will make the grid have 6 cells horizontally and 4 vertically. In particular, this is different from the subdivisions
parameter in icosphereSolid().
Trade:: MeshData3D Magnum:: Primitives:: grid3DWireframe(const Vector2i& subdivisions)
3D wireframe grid
2x2 grid. Indexed MeshPrimitive::
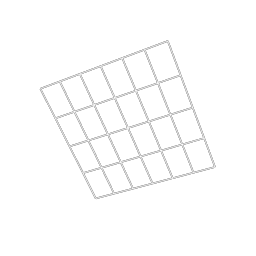
The subdivisions
parameter describes how many times the plane gets cut in each direction. Specifying {0, 0}
will make the result an (indexed) equivalent to planeWireframe(); {5, 3}
will make the grid have 6 cells horizontally and 4 vertically. In particular, this is different from the subdivisions
parameter in icosphereSolid().
Trade:: MeshData3D Magnum:: Primitives:: icosphereSolid(UnsignedInt subdivisions)
Solid 3D icosphere.
Parameters | |
---|---|
subdivisions | Number of subdivisions |
Sphere with radius 1.0f
. Indexed MeshPrimitive::
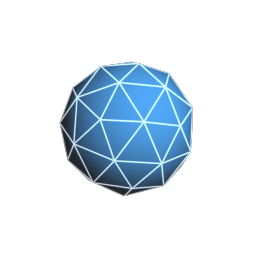
The subdivisions
parameter describes how many times is each icosphere triangle subdivided, recursively. Specifying 0
will result in an icosphere with 20 faces, saying 1
will result in an icosphere with 80 faces (each triangle subdivided into four smaller), saying 2
will result in 320 faces and so on. In particular, this is different from the subdivisions
parameter in grid3DSolid() or grid3DWireframe().
Trade:: MeshData2D Magnum:: Primitives:: line2D()
2D line
Unit-size line in direction of positive X axis. Non-indexed MeshPrimitive::
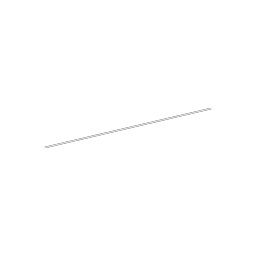
Trade:: MeshData3D Magnum:: Primitives:: line3D()
3D line
Unit-size line in direction of positive X axis. Non-indexed MeshPrimitive::
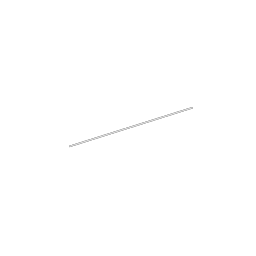
Trade:: MeshData3D Magnum:: Primitives:: planeSolid(PlaneTextureCoords textureCoords = PlaneTextureCoords:: DontGenerate)
Solid 3D plane.
2x2 plane. Non-indexed MeshPrimitive::
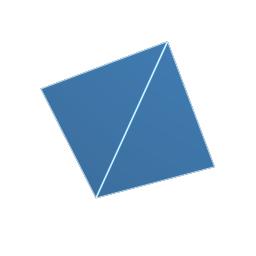
Trade:: MeshData3D Magnum:: Primitives:: planeWireframe()
Wireframe 3D plane.
2x2 plane. Non-indexed MeshPrimitive::
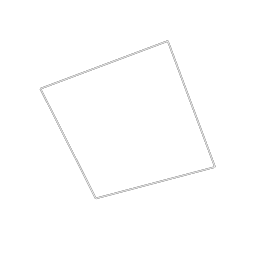
Trade:: MeshData2D Magnum:: Primitives:: squareSolid(SquareTextureCoords textureCoords = SquareTextureCoords:: DontGenerate)
Solid 2D square.
2x2 square. Non-indexed MeshPrimitive::
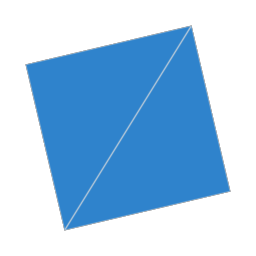
Trade:: MeshData2D Magnum:: Primitives:: squareWireframe()
Wireframe 2D square.
2x2 square. Non-indexed MeshPrimitive::
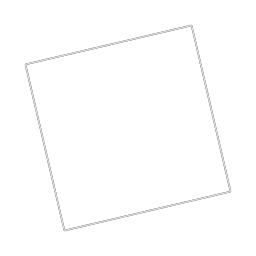
Trade:: MeshData3D Magnum:: Primitives:: uvSphereSolid(UnsignedInt rings,
UnsignedInt segments,
UVSphereTextureCoords textureCoords = UVSphereTextureCoords:: DontGenerate)
Solid 3D UV sphere.
Parameters | |
---|---|
rings | Number of (face) rings. Must be larger or equal to 2 . |
segments | Number of (face) segments. Must be larger or equal to 3 . |
textureCoords | Whether to generate texture coordinates |
Sphere with radius 1.0f
. Indexed MeshPrimitive::
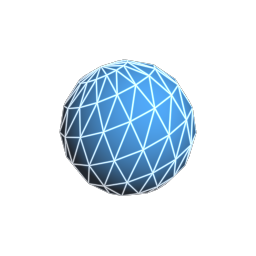
Trade:: MeshData3D Magnum:: Primitives:: uvSphereWireframe(UnsignedInt rings,
UnsignedInt segments)
Wireframe 3D UV sphere.
Parameters | |
---|---|
rings | Number of (line) rings. Must be larger or equal to 2 and multiple of 2 . |
segments | Number of (line) segments. Must be larger or equal to 4 and multiple of 4 . |
Sphere with radius 1.0f
. Indexed MeshPrimitive::
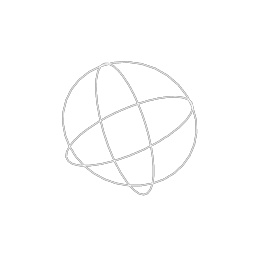